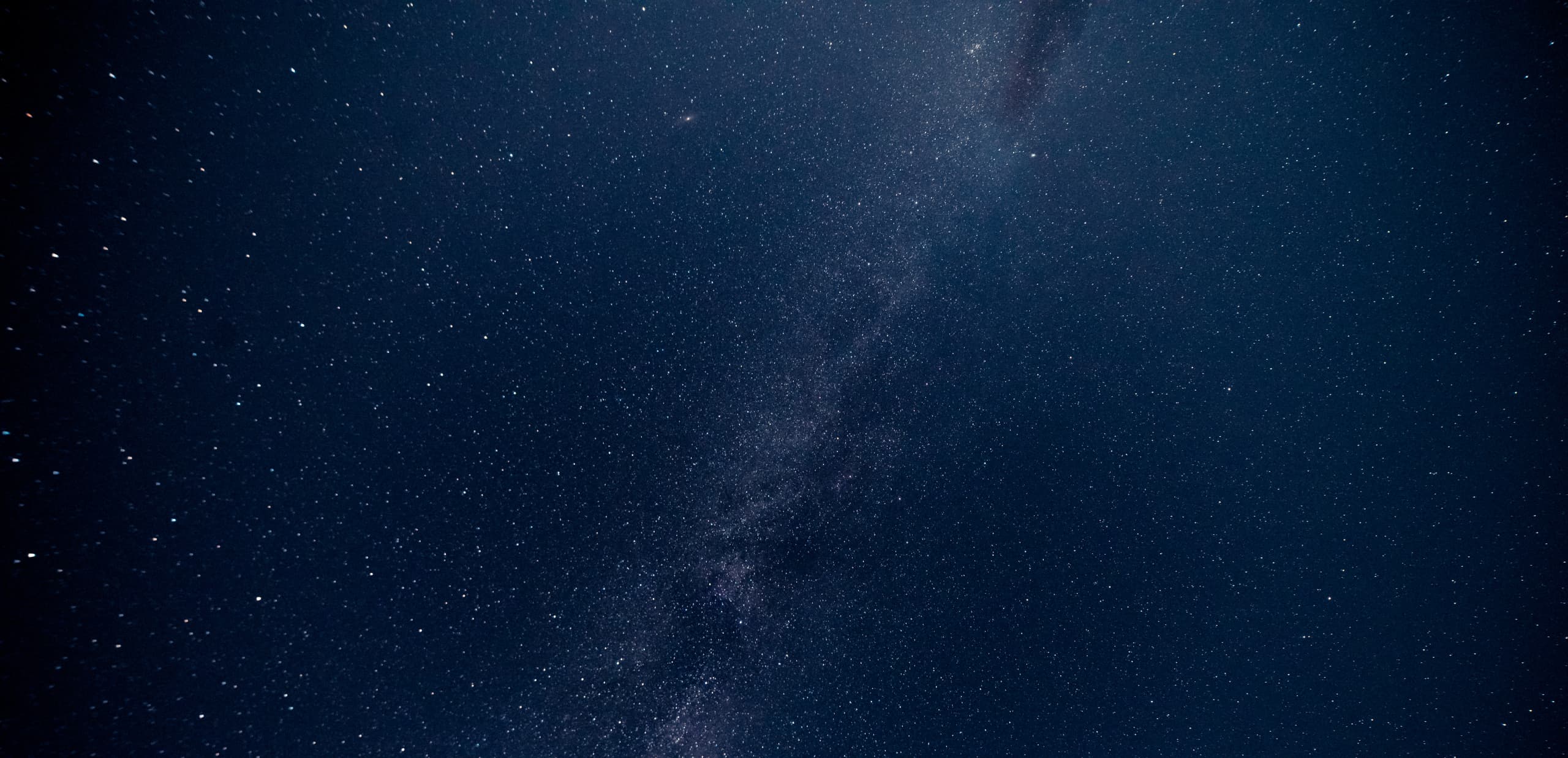
StellarDS.io
JavaScript client for StellarDataStore The Stellar DataStore service is your instantly available, secure and worry-free cloud data storage service. Sign-up now and minutes later, you can start putting your data in the cloud. This SDK is automatically generated by the OpenAPI Generator project:
- API version: v1
- Package version: v1
- Generator version: 7.10.0
- Build package: org.openapitools.codegen.languages.JavascriptClientCodegen
Installation
For Node.js
npm
Install it via:
npm install stellards-js-sdk --save
Finally, you need to build the module:
npm run build
Local development
To use the library locally without publishing to a remote npm registry, first install the dependencies by changing into the directory containing package.json
(and this README). Let's call this JAVASCRIPT_CLIENT_DIR
. Then run:
npm install
Next, link it globally in npm with the following, also from JAVASCRIPT_CLIENT_DIR
:
npm link
To use the link you just defined in your project, switch to the directory you want to use your stellards-js-sdk from, and run:
npm link /path/to/<JAVASCRIPT_CLIENT_DIR>
Finally, you need to build the module:
npm run build
git
If the library is hosted at a git repository, e.g.https://github.com/Stellar-DataStore/Javascript-sdk then install it via:
npm install Stellar-DataStore/Javascript-sdk --save
For browser
The library also works in the browser environment via npm and browserify. After following
the above steps with Node.js and installing browserify with npm install -g browserify
,
perform the following (assuming main.js is your entry file):
browserify main.js > bundle.js
Then include bundle.js in the HTML pages. Or use it directly from our server using the following code:
<script src='https://stellards.io/js/stellardssdk.js'></script>
Webpack Configuration
Using Webpack you may encounter the following error: "Module not found: Error: Cannot resolve module", most certainly you should disable AMD loader. Add/merge the following section to your webpack config:
module: {
rules: [
{
parser: {
amd: false
}
}
]
}
Getting Started
Please follow the installation instruction and execute the following JS code:
var StellarDataStore = require('stellards-js-sdk');
var defaultClient = StellarDataStore.ApiClient.instance;
// Configure API key authorization: Bearer
var Bearer = defaultClient.authentications['Bearer'];
Bearer.apiKey = "YOUR API KEY"
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//Bearer.apiKeyPrefix['Authorization'] = "Token"
var api = new StellarDataStore.DataApi()
var project = "project_example"; // {String} The project containing the table.
var table = 789; // {Number} The table containing the records.
var record = 789; // {Number} The record to be queried.
var field = "field_example"; // {String} The field of the record.
var callback = function(error, data, response) {
if (error) {
console.error(error);
} else {
console.log('API called successfully. Returned data: ' + data);
}
};
api.v1DataTableBlobGet(project, table, record, field, callback);
Documentation for API Endpoints
All URIs are relative to https://api.stellards.io
Class | Method | HTTP request | Description |
---|---|---|---|
StellarDataStore.DataApi | v1DataTableBlobGet | GET /v1/data/table/blob | Download a file from a blob field as a stream. |
StellarDataStore.DataApi | v1DataTableBlobPost | POST /v1/data/table/blob | Upload a file to a blob field. |
StellarDataStore.DataApi | v1DataTableClearDelete | DELETE /v1/data/table/clear | Deletes all records from the given table. |
StellarDataStore.DataApi | v1DataTableDelete | DELETE /v1/data/table | Deletes records from the given table. |
StellarDataStore.DataApi | v1DataTableDeletePost | POST /v1/data/table/delete | Deletes records from the given table. |
StellarDataStore.DataApi | v1DataTableGet | GET /v1/data/table | Gets the records for a given table. |
StellarDataStore.DataApi | v1DataTablePost | POST /v1/data/table | Adds records to the given table. |
StellarDataStore.DataApi | v1DataTablePut | PUT /v1/data/table | Updates records in the given table based on the values in record. |
StellarDataStore.FieldApi | v1SchemaTableFieldDelete | DELETE /v1/schema/table/field | Deletes the given field within the given table. |
StellarDataStore.FieldApi | v1SchemaTableFieldGet | GET /v1/schema/table/field | Gets field(s) from given table. |
StellarDataStore.FieldApi | v1SchemaTableFieldPost | POST /v1/schema/table/field | Adds a field to the given table. |
StellarDataStore.FieldApi | v1SchemaTableFieldPut | PUT /v1/schema/table/field | Updates the given field in the given table. |
StellarDataStore.OAuthApi | v1OauthRevokePost | POST /v1/oauth/revoke | |
StellarDataStore.OAuthApi | v1OauthTokenPost | POST /v1/oauth/token | |
StellarDataStore.ProjectApi | v1SchemaProjectGet | GET /v1/schema/project | Gets project(s) from the logged in user. |
StellarDataStore.ProjectApi | v1SchemaProjectPut | PUT /v1/schema/project | Updates a project. |
StellarDataStore.ProjectTierApi | v1ProjectTierCurrentGet | GET /v1/project-tier/current | |
StellarDataStore.ProjectTierApi | v1ProjectTierGet | GET /v1/project-tier | |
StellarDataStore.TableApi | v1SchemaTableDelete | DELETE /v1/schema/table | Deletes a table from the database. |
StellarDataStore.TableApi | v1SchemaTableGet | GET /v1/schema/table | Gets table(s) from the logged in user. |
StellarDataStore.TableApi | v1SchemaTablePost | POST /v1/schema/table | Creates a new table in the database. |
StellarDataStore.TableApi | v1SchemaTablePut | PUT /v1/schema/table | Updates a table in the database. |
StellarDataStore.TestApi | v1PingGet | GET /v1/ping | |
StellarDataStore.UserApi | v1UserDelete | DELETE /v1/user | |
StellarDataStore.UserApi | v1UserGet | GET /v1/user | |
StellarDataStore.UserApi | v1UserPermissionsGet | GET /v1/user/permissions |
Documentation for Models
- StellarDataStore.AbstractObjectQueryResult
- StellarDataStore.AbstractObjectServiceResult
- StellarDataStore.CreateRecordRequest
- StellarDataStore.FieldRequest
- StellarDataStore.FieldResponse
- StellarDataStore.FieldResponseServiceResult
- StellarDataStore.IServiceResult
- StellarDataStore.ProjectRequest
- StellarDataStore.RevokeTokenRequest
- StellarDataStore.ServiceMessage
- StellarDataStore.ServiceMessageType
- StellarDataStore.ServiceResult
- StellarDataStore.Stream
- StellarDataStore.TableRequest
- StellarDataStore.TableResponse
- StellarDataStore.TableResponseIEnumerableServiceResult
- StellarDataStore.TokenResponse
- StellarDataStore.UpdateRecordRequest
Documentation for Authorization
Authentication schemes defined for the API:
Bearer
- Type: API key
- API key parameter name: Authorization
- Location: HTTP header
StellarDataStore.AbstractObjectQueryResult
Properties
Name | Type | Description | Notes |
---|---|---|---|
messages | [ServiceMessage] | [optional] | |
isSuccess | Boolean | [optional] [readonly] | |
data | [] | [optional] | |
count | Number | [optional] |
StellarDataStore.AbstractObjectServiceResult
Properties
Name | Type | Description | Notes |
---|---|---|---|
messages | [ServiceMessage] | [optional] | |
isSuccess | Boolean | [optional] [readonly] | |
data | [optional] |
StellarDataStore.CreateRecordRequest
Properties
Name | Type | Description | Notes |
---|---|---|---|
records | [] |
StellarDataStore.DataApi
All URIs are relative to https://api.stellards.io
Method | HTTP request | Description |
---|---|---|
v1DataTableBlobGet | GET /v1/data/table/blob | Download a file from a blob field as a stream. |
v1DataTableBlobPost | POST /v1/data/table/blob | Upload a file to a blob field. |
v1DataTableClearDelete | DELETE /v1/data/table/clear | Deletes all records from the given table. |
v1DataTableDelete | DELETE /v1/data/table | Deletes records from the given table. |
v1DataTableDeletePost | POST /v1/data/table/delete | Deletes records from the given table. |
v1DataTableGet | GET /v1/data/table | Gets the records for a given table. |
v1DataTablePost | POST /v1/data/table | Adds records to the given table. |
v1DataTablePut | PUT /v1/data/table | Updates records in the given table based on the values in record. |
v1DataTableBlobGet
Stream v1DataTableBlobGet(project, table, record, field)
Download a file from a blob field as a stream.
Example
import StellarDataStore from 'stellards-js-sdk';
let defaultClient = StellarDataStore.ApiClient.instance;
// Configure API key authorization: Bearer
let Bearer = defaultClient.authentications['Bearer'];
Bearer.apiKey = 'YOUR API KEY';
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//Bearer.apiKeyPrefix = 'Token';
let apiInstance = new StellarDataStore.DataApi();
let project = "project_example"; // String | The project containing the table.
let table = 789; // Number | The table containing the records.
let record = 789; // Number | The record to be queried.
let field = "field_example"; // String | The field of the record.
apiInstance.v1DataTableBlobGet(project, table, record, field, (error, data, response) => {
if (error) {
console.error(error);
} else {
console.log('API called successfully. Returned data: ' + data);
}
});
Parameters
Name | Type | Description | Notes |
---|---|---|---|
project | String | The project containing the table. | |
table | Number | The table containing the records. | |
record | Number | The record to be queried. | |
field | String | The field of the record. |
Return type
Authorization
HTTP request headers
- Content-Type: Not defined
- Accept: application/json
v1DataTableBlobPost
Stream v1DataTableBlobPost(project, table, record, field, opts)
Upload a file to a blob field.
Example
import StellarDataStore from 'stellards-js-sdk';
let defaultClient = StellarDataStore.ApiClient.instance;
// Configure API key authorization: Bearer
let Bearer = defaultClient.authentications['Bearer'];
Bearer.apiKey = 'YOUR API KEY';
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//Bearer.apiKeyPrefix = 'Token';
let apiInstance = new StellarDataStore.DataApi();
let project = "project_example"; // String | The project containing the table.
let table = 789; // Number | The table containing the records.
let record = 789; // Number | The record to be queried.
let field = "field_example"; // String | The field of the record.
let opts = {
'data': "/path/to/file" // File |
};
apiInstance.v1DataTableBlobPost(project, table, record, field, opts, (error, data, response) => {
if (error) {
console.error(error);
} else {
console.log('API called successfully. Returned data: ' + data);
}
});
Parameters
Name | Type | Description | Notes |
---|---|---|---|
project | String | The project containing the table. | |
table | Number | The table containing the records. | |
record | Number | The record to be queried. | |
field | String | The field of the record. | |
data | File | [optional] |
Return type
Authorization
HTTP request headers
- Content-Type: multipart/form-data
- Accept: application/json
v1DataTableClearDelete
v1DataTableClearDelete(project, table)
Deletes all records from the given table.
Example
import StellarDataStore from 'stellards-js-sdk';
let defaultClient = StellarDataStore.ApiClient.instance;
// Configure API key authorization: Bearer
let Bearer = defaultClient.authentications['Bearer'];
Bearer.apiKey = 'YOUR API KEY';
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//Bearer.apiKeyPrefix = 'Token';
let apiInstance = new StellarDataStore.DataApi();
let project = "project_example"; // String | The project containing the table.
let table = 789; // Number | The table to delete from.
apiInstance.v1DataTableClearDelete(project, table, (error, data, response) => {
if (error) {
console.error(error);
} else {
console.log('API called successfully.');
}
});
Parameters
Name | Type | Description | Notes |
---|---|---|---|
project | String | The project containing the table. | |
table | Number | The table to delete from. |
Return type
null (empty response body)
Authorization
HTTP request headers
- Content-Type: Not defined
- Accept: application/json
v1DataTableDelete
v1DataTableDelete(project, table, opts)
Deletes records from the given table.
Example
import StellarDataStore from 'stellards-js-sdk';
let defaultClient = StellarDataStore.ApiClient.instance;
// Configure API key authorization: Bearer
let Bearer = defaultClient.authentications['Bearer'];
Bearer.apiKey = 'YOUR API KEY';
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//Bearer.apiKeyPrefix = 'Token';
let apiInstance = new StellarDataStore.DataApi();
let project = "project_example"; // String | The project containing the table.
let table = 789; // Number | Id of table where you want to delete a record.
let opts = {
'record': 56 // Number | record id to delete.
};
apiInstance.v1DataTableDelete(project, table, opts, (error, data, response) => {
if (error) {
console.error(error);
} else {
console.log('API called successfully.');
}
});
Parameters
Name | Type | Description | Notes |
---|---|---|---|
project | String | The project containing the table. | |
table | Number | Id of table where you want to delete a record. | |
record | Number | record id to delete. | [optional] |
Return type
null (empty response body)
Authorization
HTTP request headers
- Content-Type: Not defined
- Accept: application/json
v1DataTableDeletePost
v1DataTableDeletePost(project, table, opts)
Deletes records from the given table.
Example
import StellarDataStore from 'stellards-js-sdk';
let defaultClient = StellarDataStore.ApiClient.instance;
// Configure API key authorization: Bearer
let Bearer = defaultClient.authentications['Bearer'];
Bearer.apiKey = 'YOUR API KEY';
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//Bearer.apiKeyPrefix = 'Token';
let apiInstance = new StellarDataStore.DataApi();
let project = "project_example"; // String | The project containing the table.
let table = 56; // Number | The table to delete from.
let opts = {
'requestBody': ["null"] // [String] | An array of ids
};
apiInstance.v1DataTableDeletePost(project, table, opts, (error, data, response) => {
if (error) {
console.error(error);
} else {
console.log('API called successfully.');
}
});
Parameters
Name | Type | Description | Notes |
---|---|---|---|
project | String | The project containing the table. | |
table | Number | The table to delete from. | |
requestBody | [String] | An array of ids | [optional] |
Return type
null (empty response body)
Authorization
HTTP request headers
- Content-Type: application/json, text/json, application/*+json
- Accept: application/json
v1DataTableGet
AbstractObjectQueryResult v1DataTableGet(project, table, opts)
Gets the records for a given table.
Example
import StellarDataStore from 'stellards-js-sdk';
let defaultClient = StellarDataStore.ApiClient.instance;
// Configure API key authorization: Bearer
let Bearer = defaultClient.authentications['Bearer'];
Bearer.apiKey = 'YOUR API KEY';
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//Bearer.apiKeyPrefix = 'Token';
let apiInstance = new StellarDataStore.DataApi();
let project = "project_example"; // String | The project containing the table.
let table = 789; // Number | Id of the table containing the records.
let opts = {
'offset': 789, // Number | The offset of the records.
'take': 789, // Number | The amount of records.
'joinQuery': "joinQuery_example", // String | The join queries to apply.
'whereQuery': "whereQuery_example", // String | The where queries to apply.
'sortQuery': "sortQuery_example", // String | The sort queries to apply.
'distinct': false, // Boolean |
'select': "select_example" // String |
};
apiInstance.v1DataTableGet(project, table, opts, (error, data, response) => {
if (error) {
console.error(error);
} else {
console.log('API called successfully. Returned data: ' + data);
}
});
Parameters
Name | Type | Description | Notes |
---|---|---|---|
project | String | The project containing the table. | |
table | Number | Id of the table containing the records. | |
offset | Number | The offset of the records. | [optional] |
take | Number | The amount of records. | [optional] |
joinQuery | String | The join queries to apply. | [optional] |
whereQuery | String | The where queries to apply. | [optional] |
sortQuery | String | The sort queries to apply. | [optional] |
distinct | Boolean | [optional] [default to false] | |
select | String | [optional] |
Return type
Authorization
HTTP request headers
- Content-Type: Not defined
- Accept: application/json
v1DataTablePost
AbstractObjectQueryResult v1DataTablePost(project, table, opts)
Adds records to the given table.
Example
import StellarDataStore from 'stellards-js-sdk';
let defaultClient = StellarDataStore.ApiClient.instance;
// Configure API key authorization: Bearer
let Bearer = defaultClient.authentications['Bearer'];
Bearer.apiKey = 'YOUR API KEY';
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//Bearer.apiKeyPrefix = 'Token';
let apiInstance = new StellarDataStore.DataApi();
let project = "project_example"; // String | The project containing the table.
let table = 789; // Number | The table to be added to.
let opts = {
'createRecordRequest': new StellarDataStore.CreateRecordRequest() // CreateRecordRequest |
};
apiInstance.v1DataTablePost(project, table, opts, (error, data, response) => {
if (error) {
console.error(error);
} else {
console.log('API called successfully. Returned data: ' + data);
}
});
Parameters
Name | Type | Description | Notes |
---|---|---|---|
project | String | The project containing the table. | |
table | Number | The table to be added to. | |
createRecordRequest | CreateRecordRequest | [optional] |
Return type
Authorization
HTTP request headers
- Content-Type: application/json, text/json, application/*+json
- Accept: application/json
v1DataTablePut
AbstractObjectQueryResult v1DataTablePut(project, table, opts)
Updates records in the given table based on the values in record.
Example
import StellarDataStore from 'stellards-js-sdk';
let defaultClient = StellarDataStore.ApiClient.instance;
// Configure API key authorization: Bearer
let Bearer = defaultClient.authentications['Bearer'];
Bearer.apiKey = 'YOUR API KEY';
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//Bearer.apiKeyPrefix = 'Token';
let apiInstance = new StellarDataStore.DataApi();
let project = "project_example"; // String | The project containing the table.
let table = 789; // Number | The table to be updated in.
let opts = {
'force': false, // Boolean | If you are certain you want to update all your records in case no ids were included.
'updateRecordRequest': new StellarDataStore.UpdateRecordRequest() // UpdateRecordRequest |
};
apiInstance.v1DataTablePut(project, table, opts, (error, data, response) => {
if (error) {
console.error(error);
} else {
console.log('API called successfully. Returned data: ' + data);
}
});
Parameters
Name | Type | Description | Notes |
---|---|---|---|
project | String | The project containing the table. | |
table | Number | The table to be updated in. | |
force | Boolean | If you are certain you want to update all your records in case no ids were included. | [optional] [default to false] |
updateRecordRequest | UpdateRecordRequest | [optional] |
Return type
Authorization
HTTP request headers
- Content-Type: application/json, text/json, application/*+json
- Accept: application/json
StellarDataStore.FieldApi
All URIs are relative to https://api.stellards.io
Method | HTTP request | Description |
---|---|---|
v1SchemaTableFieldDelete | DELETE /v1/schema/table/field | Deletes the given field within the given table. |
v1SchemaTableFieldGet | GET /v1/schema/table/field | Gets field(s) from given table. |
v1SchemaTableFieldPost | POST /v1/schema/table/field | Adds a field to the given table. |
v1SchemaTableFieldPut | PUT /v1/schema/table/field | Updates the given field in the given table. |
v1SchemaTableFieldDelete
v1SchemaTableFieldDelete(project, table, field)
Deletes the given field within the given table.
Example
import StellarDataStore from 'stellards-js-sdk';
let defaultClient = StellarDataStore.ApiClient.instance;
// Configure API key authorization: Bearer
let Bearer = defaultClient.authentications['Bearer'];
Bearer.apiKey = 'YOUR API KEY';
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//Bearer.apiKeyPrefix = 'Token';
let apiInstance = new StellarDataStore.FieldApi();
let project = "project_example"; // String | The project containing the table.
let table = 56; // Number | The table containing the given field.
let field = 56; // Number | The field to be deleted
apiInstance.v1SchemaTableFieldDelete(project, table, field, (error, data, response) => {
if (error) {
console.error(error);
} else {
console.log('API called successfully.');
}
});
Parameters
Name | Type | Description | Notes |
---|---|---|---|
project | String | The project containing the table. | |
table | Number | The table containing the given field. | |
field | Number | The field to be deleted |
Return type
null (empty response body)
Authorization
HTTP request headers
- Content-Type: Not defined
- Accept: text/plain, application/json, text/json
v1SchemaTableFieldGet
IServiceResult v1SchemaTableFieldGet(project, table, opts)
Gets field(s) from given table.
Example
import StellarDataStore from 'stellards-js-sdk';
let defaultClient = StellarDataStore.ApiClient.instance;
// Configure API key authorization: Bearer
let Bearer = defaultClient.authentications['Bearer'];
Bearer.apiKey = 'YOUR API KEY';
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//Bearer.apiKeyPrefix = 'Token';
let apiInstance = new StellarDataStore.FieldApi();
let project = "project_example"; // String | The project containing the table.
let table = 56; // Number | The table containing the fields.
let opts = {
'field': 56 // Number | The optional field to get.
};
apiInstance.v1SchemaTableFieldGet(project, table, opts, (error, data, response) => {
if (error) {
console.error(error);
} else {
console.log('API called successfully. Returned data: ' + data);
}
});
Parameters
Name | Type | Description | Notes |
---|---|---|---|
project | String | The project containing the table. | |
table | Number | The table containing the fields. | |
field | Number | The optional field to get. | [optional] |
Return type
Authorization
HTTP request headers
- Content-Type: Not defined
- Accept: text/plain, application/json, text/json
v1SchemaTableFieldPost
FieldResponseServiceResult v1SchemaTableFieldPost(project, table, opts)
Adds a field to the given table.
Example
import StellarDataStore from 'stellards-js-sdk';
let defaultClient = StellarDataStore.ApiClient.instance;
// Configure API key authorization: Bearer
let Bearer = defaultClient.authentications['Bearer'];
Bearer.apiKey = 'YOUR API KEY';
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//Bearer.apiKeyPrefix = 'Token';
let apiInstance = new StellarDataStore.FieldApi();
let project = "project_example"; // String | The project containing the table.
let table = 56; // Number | The table to be added to.
let opts = {
'fieldRequest': new StellarDataStore.FieldRequest() // FieldRequest |
};
apiInstance.v1SchemaTableFieldPost(project, table, opts, (error, data, response) => {
if (error) {
console.error(error);
} else {
console.log('API called successfully. Returned data: ' + data);
}
});
Parameters
Name | Type | Description | Notes |
---|---|---|---|
project | String | The project containing the table. | |
table | Number | The table to be added to. | |
fieldRequest | FieldRequest | [optional] |
Return type
Authorization
HTTP request headers
- Content-Type: application/json, text/json, application/*+json
- Accept: text/plain, application/json, text/json
v1SchemaTableFieldPut
FieldResponseServiceResult v1SchemaTableFieldPut(project, table, field, opts)
Updates the given field in the given table.
Example
import StellarDataStore from 'stellards-js-sdk';
let defaultClient = StellarDataStore.ApiClient.instance;
// Configure API key authorization: Bearer
let Bearer = defaultClient.authentications['Bearer'];
Bearer.apiKey = 'YOUR API KEY';
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//Bearer.apiKeyPrefix = 'Token';
let apiInstance = new StellarDataStore.FieldApi();
let project = "project_example"; // String | The project containing the table.
let table = 56; // Number | The table containing the field.
let field = 56; // Number | The field to be updated.
let opts = {
'fieldRequest': new StellarDataStore.FieldRequest() // FieldRequest |
};
apiInstance.v1SchemaTableFieldPut(project, table, field, opts, (error, data, response) => {
if (error) {
console.error(error);
} else {
console.log('API called successfully. Returned data: ' + data);
}
});
Parameters
Name | Type | Description | Notes |
---|---|---|---|
project | String | The project containing the table. | |
table | Number | The table containing the field. | |
field | Number | The field to be updated. | |
fieldRequest | FieldRequest | [optional] |
Return type
Authorization
HTTP request headers
- Content-Type: application/json, text/json, application/*+json
- Accept: text/plain, application/json, text/json
StellarDataStore.FieldRequest
Properties
Name | Type | Description | Notes |
---|---|---|---|
name | String | ||
type | String |
StellarDataStore.FieldResponse
Properties
Name | Type | Description | Notes |
---|---|---|---|
id | Number | [optional] | |
name | String | [optional] | |
type | String | [optional] |
StellarDataStore.FieldResponseServiceResult
Properties
Name | Type | Description | Notes |
---|---|---|---|
messages | [ServiceMessage] | [optional] | |
isSuccess | Boolean | [optional] [readonly] | |
data | FieldResponse | [optional] |
StellarDataStore.IServiceResult
Properties
Name | Type | Description | Notes |
---|---|---|---|
isSuccess | Boolean | [optional] [readonly] | |
messages | [ServiceMessage] | [optional] [readonly] |
StellarDataStore.OAuthApi
All URIs are relative to https://api.stellards.io
Method | HTTP request | Description |
---|---|---|
v1OauthRevokePost | POST /v1/oauth/revoke | |
v1OauthTokenPost | POST /v1/oauth/token |
v1OauthRevokePost
v1OauthRevokePost(revokeTokenRequest)
Example
import StellarDataStore from 'stellards-js-sdk';
let defaultClient = StellarDataStore.ApiClient.instance;
// Configure API key authorization: Bearer
let Bearer = defaultClient.authentications['Bearer'];
Bearer.apiKey = 'YOUR API KEY';
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//Bearer.apiKeyPrefix = 'Token';
let apiInstance = new StellarDataStore.OAuthApi();
let revokeTokenRequest = new StellarDataStore.RevokeTokenRequest(); // RevokeTokenRequest |
apiInstance.v1OauthRevokePost(revokeTokenRequest, (error, data, response) => {
if (error) {
console.error(error);
} else {
console.log('API called successfully.');
}
});
Parameters
Name | Type | Description | Notes |
---|---|---|---|
revokeTokenRequest | RevokeTokenRequest |
Return type
null (empty response body)
Authorization
HTTP request headers
- Content-Type: application/json, text/json, application/*+json
- Accept: Not defined
v1OauthTokenPost
TokenResponse v1OauthTokenPost(grantType, clientId, clientSecret, opts)
Example
import StellarDataStore from 'stellards-js-sdk';
let defaultClient = StellarDataStore.ApiClient.instance;
// Configure API key authorization: Bearer
let Bearer = defaultClient.authentications['Bearer'];
Bearer.apiKey = 'YOUR API KEY';
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//Bearer.apiKeyPrefix = 'Token';
let apiInstance = new StellarDataStore.OAuthApi();
let grantType = "grantType_example"; // String |
let clientId = "clientId_example"; // String |
let clientSecret = "clientSecret_example"; // String |
let opts = {
'redirectUri': "''", // String |
'code': "''", // String |
'refreshToken': "''" // String |
};
apiInstance.v1OauthTokenPost(grantType, clientId, clientSecret, opts, (error, data, response) => {
if (error) {
console.error(error);
} else {
console.log('API called successfully. Returned data: ' + data);
}
});
Parameters
Name | Type | Description | Notes |
---|---|---|---|
grantType | String | ||
clientId | String | ||
clientSecret | String | ||
redirectUri | String | [optional] [default to ''] | |
code | String | [optional] [default to ''] | |
refreshToken | String | [optional] [default to ''] |
Return type
Authorization
HTTP request headers
- Content-Type: application/x-www-form-urlencoded
- Accept: application/json
StellarDataStore.ProjectApi
All URIs are relative to https://api.stellards.io
Method | HTTP request | Description |
---|---|---|
v1SchemaProjectGet | GET /v1/schema/project | Gets project(s) from the logged in user. |
v1SchemaProjectPut | PUT /v1/schema/project | Updates a project. |
v1SchemaProjectGet
IServiceResult v1SchemaProjectGet(opts)
Gets project(s) from the logged in user.
Example
import StellarDataStore from 'stellards-js-sdk';
let defaultClient = StellarDataStore.ApiClient.instance;
// Configure API key authorization: Bearer
let Bearer = defaultClient.authentications['Bearer'];
Bearer.apiKey = 'YOUR API KEY';
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//Bearer.apiKeyPrefix = 'Token';
let apiInstance = new StellarDataStore.ProjectApi();
let opts = {
'project': "project_example" // String | The optional project guid to be fetched.
};
apiInstance.v1SchemaProjectGet(opts, (error, data, response) => {
if (error) {
console.error(error);
} else {
console.log('API called successfully. Returned data: ' + data);
}
});
Parameters
Name | Type | Description | Notes |
---|---|---|---|
project | String | The optional project guid to be fetched. | [optional] |
Return type
Authorization
HTTP request headers
- Content-Type: Not defined
- Accept: text/plain, application/json, text/json
v1SchemaProjectPut
TableResponseIEnumerableServiceResult v1SchemaProjectPut(project, opts)
Updates a project.
Example
import StellarDataStore from 'stellards-js-sdk';
let defaultClient = StellarDataStore.ApiClient.instance;
// Configure API key authorization: Bearer
let Bearer = defaultClient.authentications['Bearer'];
Bearer.apiKey = 'YOUR API KEY';
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//Bearer.apiKeyPrefix = 'Token';
let apiInstance = new StellarDataStore.ProjectApi();
let project = "project_example"; // String | The project to be updated.
let opts = {
'projectRequest': new StellarDataStore.ProjectRequest() // ProjectRequest |
};
apiInstance.v1SchemaProjectPut(project, opts, (error, data, response) => {
if (error) {
console.error(error);
} else {
console.log('API called successfully. Returned data: ' + data);
}
});
Parameters
Name | Type | Description | Notes |
---|---|---|---|
project | String | The project to be updated. | |
projectRequest | ProjectRequest | [optional] |
Return type
TableResponseIEnumerableServiceResult
Authorization
HTTP request headers
- Content-Type: application/json, text/json, application/*+json
- Accept: text/plain, application/json, text/json
StellarDataStore.ProjectRequest
Properties
Name | Type | Description | Notes |
---|---|---|---|
name | String | ||
description | String | [optional] | |
isMultitenant | Boolean | ||
allowNewUsers | Boolean |
StellarDataStore.ProjectTierApi
All URIs are relative to https://api.stellards.io
Method | HTTP request | Description |
---|---|---|
v1ProjectTierCurrentGet | GET /v1/project-tier/current | |
v1ProjectTierGet | GET /v1/project-tier |
v1ProjectTierCurrentGet
IServiceResult v1ProjectTierCurrentGet(project)
Example
import StellarDataStore from 'stellards-js-sdk';
let defaultClient = StellarDataStore.ApiClient.instance;
// Configure API key authorization: Bearer
let Bearer = defaultClient.authentications['Bearer'];
Bearer.apiKey = 'YOUR API KEY';
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//Bearer.apiKeyPrefix = 'Token';
let apiInstance = new StellarDataStore.ProjectTierApi();
let project = "project_example"; // String |
apiInstance.v1ProjectTierCurrentGet(project, (error, data, response) => {
if (error) {
console.error(error);
} else {
console.log('API called successfully. Returned data: ' + data);
}
});
Parameters
Name | Type | Description | Notes |
---|---|---|---|
project | String |
Return type
Authorization
HTTP request headers
- Content-Type: Not defined
- Accept: text/plain, application/json, text/json
v1ProjectTierGet
IServiceResult v1ProjectTierGet(project)
Example
import StellarDataStore from 'stellards-js-sdk';
let defaultClient = StellarDataStore.ApiClient.instance;
// Configure API key authorization: Bearer
let Bearer = defaultClient.authentications['Bearer'];
Bearer.apiKey = 'YOUR API KEY';
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//Bearer.apiKeyPrefix = 'Token';
let apiInstance = new StellarDataStore.ProjectTierApi();
let project = "project_example"; // String |
apiInstance.v1ProjectTierGet(project, (error, data, response) => {
if (error) {
console.error(error);
} else {
console.log('API called successfully. Returned data: ' + data);
}
});
Parameters
Name | Type | Description | Notes |
---|---|---|---|
project | String |
Return type
Authorization
HTTP request headers
- Content-Type: Not defined
- Accept: text/plain, application/json, text/json
StellarDataStore.RevokeTokenRequest
Properties
Name | Type | Description | Notes |
---|---|---|---|
refreshToken | String | ||
clientId | String | ||
clientSecret | String |
StellarDataStore.ServiceMessage
Properties
Name | Type | Description | Notes |
---|---|---|---|
code | String | [optional] | |
message | String | [optional] | |
type | ServiceMessageType | [optional] |
StellarDataStore.ServiceMessageType
Enum
10
(value:10
)20
(value:20
)30
(value:30
)31
(value:31
)32
(value:32
)
StellarDataStore.ServiceResult
Properties
Name | Type | Description | Notes |
---|---|---|---|
messages | [ServiceMessage] | [optional] | |
isSuccess | Boolean | [optional] [readonly] |
StellarDataStore.Stream
Properties
Name | Type | Description | Notes |
---|---|---|---|
canRead | Boolean | [optional] [readonly] | |
canWrite | Boolean | [optional] [readonly] | |
canSeek | Boolean | [optional] [readonly] | |
canTimeout | Boolean | [optional] [readonly] | |
length | Number | [optional] [readonly] | |
position | Number | [optional] | |
readTimeout | Number | [optional] | |
writeTimeout | Number | [optional] |
StellarDataStore.TableApi
All URIs are relative to https://api.stellards.io
Method | HTTP request | Description |
---|---|---|
v1SchemaTableDelete | DELETE /v1/schema/table | Deletes a table from the database. |
v1SchemaTableGet | GET /v1/schema/table | Gets table(s) from the logged in user. |
v1SchemaTablePost | POST /v1/schema/table | Creates a new table in the database. |
v1SchemaTablePut | PUT /v1/schema/table | Updates a table in the database. |
v1SchemaTableDelete
v1SchemaTableDelete(project, table)
Deletes a table from the database.
Example
import StellarDataStore from 'stellards-js-sdk';
let defaultClient = StellarDataStore.ApiClient.instance;
// Configure API key authorization: Bearer
let Bearer = defaultClient.authentications['Bearer'];
Bearer.apiKey = 'YOUR API KEY';
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//Bearer.apiKeyPrefix = 'Token';
let apiInstance = new StellarDataStore.TableApi();
let project = "project_example"; // String | The project containing the table.
let table = 56; // Number | The table to be deleted.
apiInstance.v1SchemaTableDelete(project, table, (error, data, response) => {
if (error) {
console.error(error);
} else {
console.log('API called successfully.');
}
});
Parameters
Name | Type | Description | Notes |
---|---|---|---|
project | String | The project containing the table. | |
table | Number | The table to be deleted. |
Return type
null (empty response body)
Authorization
HTTP request headers
- Content-Type: Not defined
- Accept: text/plain, application/json, text/json
v1SchemaTableGet
IServiceResult v1SchemaTableGet(project, opts)
Gets table(s) from the logged in user.
Example
import StellarDataStore from 'stellards-js-sdk';
let defaultClient = StellarDataStore.ApiClient.instance;
// Configure API key authorization: Bearer
let Bearer = defaultClient.authentications['Bearer'];
Bearer.apiKey = 'YOUR API KEY';
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//Bearer.apiKeyPrefix = 'Token';
let apiInstance = new StellarDataStore.TableApi();
let project = "project_example"; // String | The project containing the table.
let opts = {
'table': "table_example" // String | name or id of the table to fetch (optional)
};
apiInstance.v1SchemaTableGet(project, opts, (error, data, response) => {
if (error) {
console.error(error);
} else {
console.log('API called successfully. Returned data: ' + data);
}
});
Parameters
Name | Type | Description | Notes |
---|---|---|---|
project | String | The project containing the table. | |
table | String | name or id of the table to fetch (optional) | [optional] |
Return type
Authorization
HTTP request headers
- Content-Type: Not defined
- Accept: text/plain, application/json, text/json
v1SchemaTablePost
AbstractObjectServiceResult v1SchemaTablePost(project, opts)
Creates a new table in the database.
Example
import StellarDataStore from 'stellards-js-sdk';
let defaultClient = StellarDataStore.ApiClient.instance;
// Configure API key authorization: Bearer
let Bearer = defaultClient.authentications['Bearer'];
Bearer.apiKey = 'YOUR API KEY';
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//Bearer.apiKeyPrefix = 'Token';
let apiInstance = new StellarDataStore.TableApi();
let project = "project_example"; // String | The project containing the table.
let opts = {
'tableRequest': new StellarDataStore.TableRequest() // TableRequest |
};
apiInstance.v1SchemaTablePost(project, opts, (error, data, response) => {
if (error) {
console.error(error);
} else {
console.log('API called successfully. Returned data: ' + data);
}
});
Parameters
Name | Type | Description | Notes |
---|---|---|---|
project | String | The project containing the table. | |
tableRequest | TableRequest | [optional] |
Return type
Authorization
HTTP request headers
- Content-Type: application/json, text/json, application/*+json
- Accept: text/plain, application/json, text/json
v1SchemaTablePut
TableResponseIEnumerableServiceResult v1SchemaTablePut(project, opts)
Updates a table in the database.
Example
import StellarDataStore from 'stellards-js-sdk';
let defaultClient = StellarDataStore.ApiClient.instance;
// Configure API key authorization: Bearer
let Bearer = defaultClient.authentications['Bearer'];
Bearer.apiKey = 'YOUR API KEY';
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//Bearer.apiKeyPrefix = 'Token';
let apiInstance = new StellarDataStore.TableApi();
let project = "project_example"; // String | The project containing the table.
let opts = {
'table': 56, // Number | The table to be updated.
'tableRequest': new StellarDataStore.TableRequest() // TableRequest |
};
apiInstance.v1SchemaTablePut(project, opts, (error, data, response) => {
if (error) {
console.error(error);
} else {
console.log('API called successfully. Returned data: ' + data);
}
});
Parameters
Name | Type | Description | Notes |
---|---|---|---|
project | String | The project containing the table. | |
table | Number | The table to be updated. | [optional] |
tableRequest | TableRequest | [optional] |
Return type
TableResponseIEnumerableServiceResult
Authorization
HTTP request headers
- Content-Type: application/json, text/json, application/*+json
- Accept: text/plain, application/json, text/json
StellarDataStore.TableRequest
Properties
Name | Type | Description | Notes |
---|---|---|---|
name | String | ||
description | String | [optional] | |
isMultitenant | Boolean |
StellarDataStore.TableResponse
Properties
Name | Type | Description | Notes |
---|---|---|---|
id | Number | [optional] | |
name | String | [optional] | |
description | String | [optional] | |
isMultitenant | Boolean | [optional] |
StellarDataStore.TableResponseIEnumerableServiceResult
Properties
Name | Type | Description | Notes |
---|---|---|---|
messages | [ServiceMessage] | [optional] | |
isSuccess | Boolean | [optional] [readonly] | |
data | [TableResponse] | [optional] |
StellarDataStore.TestApi
All URIs are relative to https://api.stellards.io
Method | HTTP request | Description |
---|---|---|
v1PingGet | GET /v1/ping |
v1PingGet
v1PingGet()
Example
import StellarDataStore from 'stellards-js-sdk';
let defaultClient = StellarDataStore.ApiClient.instance;
// Configure API key authorization: Bearer
let Bearer = defaultClient.authentications['Bearer'];
Bearer.apiKey = 'YOUR API KEY';
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//Bearer.apiKeyPrefix = 'Token';
let apiInstance = new StellarDataStore.TestApi();
apiInstance.v1PingGet((error, data, response) => {
if (error) {
console.error(error);
} else {
console.log('API called successfully.');
}
});
Parameters
This endpoint does not need any parameter.
Return type
null (empty response body)
Authorization
HTTP request headers
- Content-Type: Not defined
- Accept: Not defined
StellarDataStore.TokenResponse
Properties
Name | Type | Description | Notes |
---|---|---|---|
accessToken | String | [optional] | |
refreshToken | String | [optional] | |
expiresIn | Number | [optional] | |
tokenType | String | [optional] |
StellarDataStore.UpdateRecordRequest
Properties
Name | Type | Description | Notes |
---|---|---|---|
idList | [Object] | [optional] | |
record |
StellarDataStore.UserApi
All URIs are relative to https://api.stellards.io
Method | HTTP request | Description |
---|---|---|
v1UserDelete | DELETE /v1/user | |
v1UserGet | GET /v1/user | |
v1UserPermissionsGet | GET /v1/user/permissions |
v1UserDelete
IServiceResult v1UserDelete()
Example
import StellarDataStore from 'stellards-js-sdk';
let defaultClient = StellarDataStore.ApiClient.instance;
// Configure API key authorization: Bearer
let Bearer = defaultClient.authentications['Bearer'];
Bearer.apiKey = 'YOUR API KEY';
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//Bearer.apiKeyPrefix = 'Token';
let apiInstance = new StellarDataStore.UserApi();
apiInstance.v1UserDelete((error, data, response) => {
if (error) {
console.error(error);
} else {
console.log('API called successfully. Returned data: ' + data);
}
});
Parameters
This endpoint does not need any parameter.
Return type
Authorization
HTTP request headers
- Content-Type: Not defined
- Accept: text/plain, application/json, text/json
v1UserGet
IServiceResult v1UserGet()
Example
import StellarDataStore from 'stellards-js-sdk';
let defaultClient = StellarDataStore.ApiClient.instance;
// Configure API key authorization: Bearer
let Bearer = defaultClient.authentications['Bearer'];
Bearer.apiKey = 'YOUR API KEY';
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//Bearer.apiKeyPrefix = 'Token';
let apiInstance = new StellarDataStore.UserApi();
apiInstance.v1UserGet((error, data, response) => {
if (error) {
console.error(error);
} else {
console.log('API called successfully. Returned data: ' + data);
}
});
Parameters
This endpoint does not need any parameter.
Return type
Authorization
HTTP request headers
- Content-Type: Not defined
- Accept: text/plain, application/json, text/json
v1UserPermissionsGet
IServiceResult v1UserPermissionsGet(projectId)
Example
import StellarDataStore from 'stellards-js-sdk';
let defaultClient = StellarDataStore.ApiClient.instance;
// Configure API key authorization: Bearer
let Bearer = defaultClient.authentications['Bearer'];
Bearer.apiKey = 'YOUR API KEY';
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//Bearer.apiKeyPrefix = 'Token';
let apiInstance = new StellarDataStore.UserApi();
let projectId = "projectId_example"; // String |
apiInstance.v1UserPermissionsGet(projectId, (error, data, response) => {
if (error) {
console.error(error);
} else {
console.log('API called successfully. Returned data: ' + data);
}
});
Parameters
Name | Type | Description | Notes |
---|---|---|---|
projectId | String |
Return type
Authorization
HTTP request headers
- Content-Type: Not defined
- Accept: text/plain, application/json, text/json